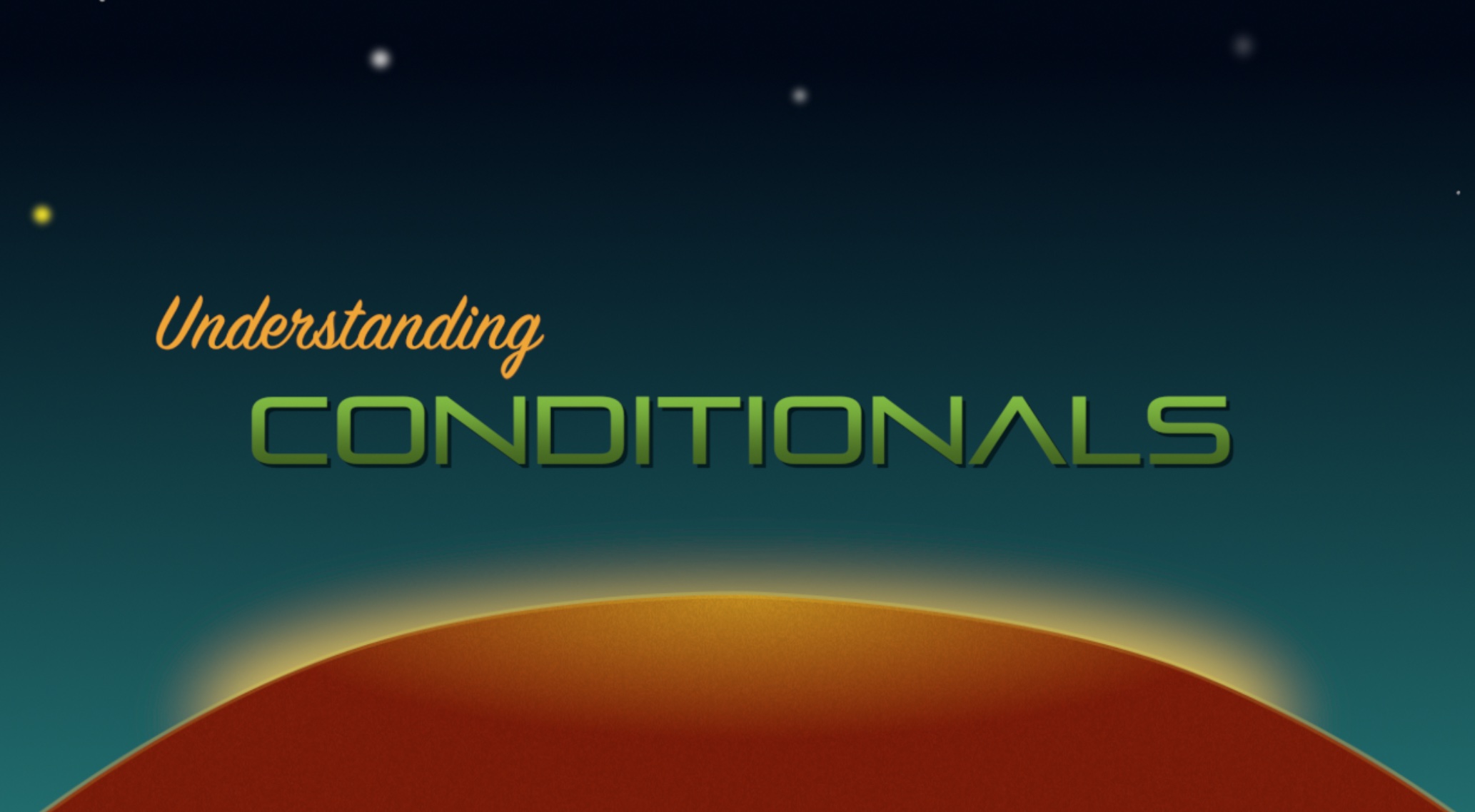
Here's something weird to think about: in a high-level functional language such as Elixir, writing conditional code is not exactly idiomatic. Using a combination of pattern matching and syntax rules we can write extremely clean code free of if statements and conditionals in general
One thing I dislike about most code books is they tell you about syntax rules, but not about how people actually write the code in the wild. I need you to write solid code here at Red:4, so let's take a second to talk about style.
If You Need "if"
Let's revisit our Solar
module's power
function. When I first wrote it, I did what most programmers would do:
defmodule Solar do
def power(flare) do
factor = 1
if(flare.classification == :M) do
factor = 10
end
if(flare.classification == :X) do
factor = 1000
end
factor * flare.scale
end
end
This code isn't bad, necessarily, but you'll notice the lack of else if
here. Which is OK, as that's basically the same thing as a bunch of if
statements.
The interesting thing about if
(and unless
) in Elixir is that they're not really a part of the language - they're macros:
An interesting note regarding if/2 and unless/2 is that they are implemented as macros in the language; they aren’t special language constructs as they would be in many languages. You can check the documentation and the source of if/2 in the Kernel module docs...
An if
(or unless
) statement is generally only used when you have a single condition you want to evaluate and they aren't used very often. In fact, I've written a number of Elixir libraries (some quite large) as well as a pretty large ecommerce application. I have, perhaps, two if
statements (if that) in all of the Elixir code I've ever written.
You just don't need them when you have pattern matching and smaller, isolated functions.