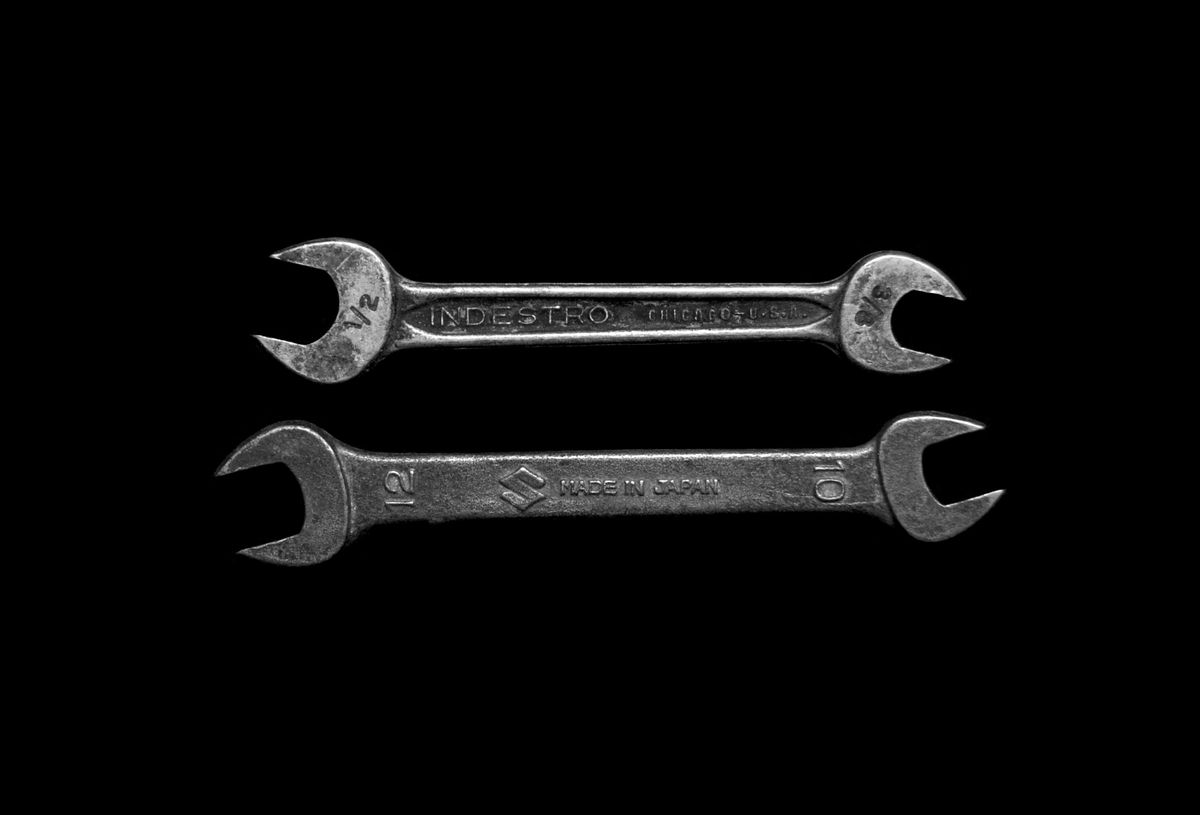
Using Make to Improve Your Test Suite
This is a premium course, which you can purchase below.
No video with this one - just a post with lots of code. Make has been around forever and is often overlooked in favor of tools that recreate precisely what it does, but in crappier ways. Let's see how you can use Make to help your testing process.
null