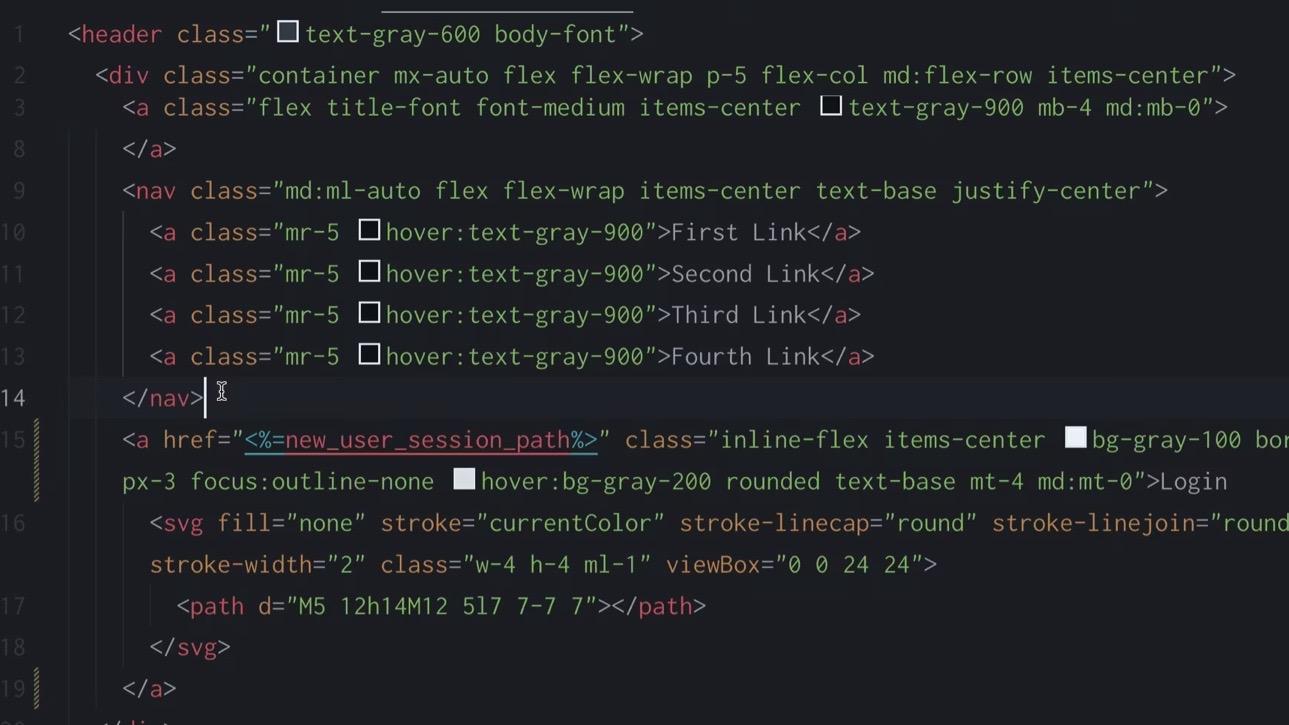
Logging Out and Registering On the Fly
This is a premium course, which you can purchase below.
Let's see how to logout with a single click, and what might get in our way. We'll also ensure that users can register on the fly.
Rails is helpful when it comes to providing a positive experience for your user, and one of those helpful things is to not make any changes with a simple GET request, such as logging someone out. To that end, we need to figure out how we can comply with this notion without writing a bunch of code! Thankfully, it's straightforward:
<%= button_to "Logout", destroy_user_session_path, method: :delete, data: {turbo: false} %>
OK, maybe that's not entirely straightforward! We have to use button_to
because the link_to
helper will only create an anchor
tag, which fires a GET
request. The button_to
helper will create a form for us with the correct method. By adding data: {turbo: false}
, we're also turning off turbo links, which can mess with the form submission.
The next task is overriding the base controller with our own (controllers/users/passwordless_controller.rb
):
class Users::PasswordlessController < Devise::Passwordless::SessionsController
def create
if params[:user].nil? || params[:user][:email].nil?
return redirect_to new_user_session_path, alert: "Please enter an email address"
end
user = User.find_by(email: params[:user][:email])
unless user
user = User.create(email: params[:user][:email])
end
user.send_magic_link
redirect_to new_user_session_path, notice: "Magic link sent!"
end
end